We often require establishing communication between components in vue js to pass the data between two or more components or just to set data flags in another component to change the state of application or specific component.
We can achieve this feature using Eventbus. Eventbus is a way to communicate between components using an observer pattern, where one component subscribes for a particular event to happen and another one triggers/fires/notifies the event listener.
Ex. Suppose we have two components C1 and C2. C2 want to call method of C1
Graphical representation (Communication between components in vue js):
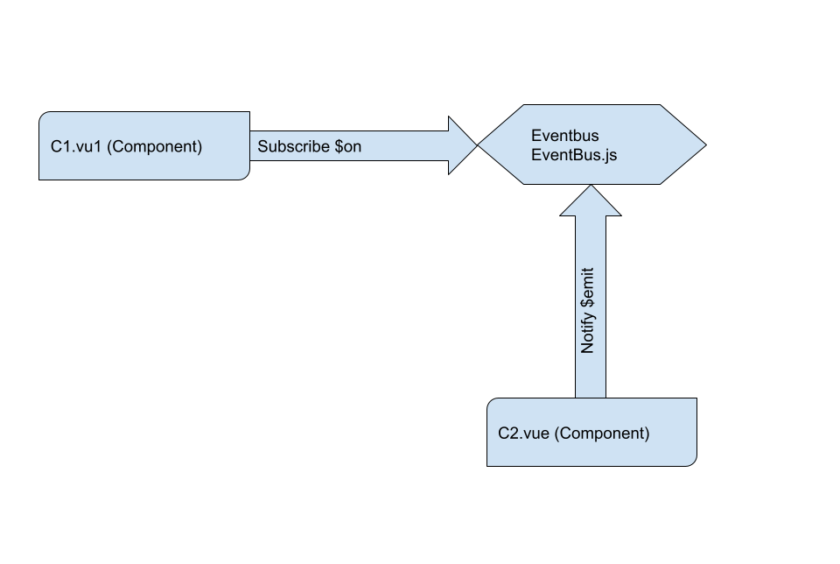
Now, let’s see how to use Eventbus in vue js application, you can also click on the following link to try it yourself:
Step1: Create EventBus.js file
import Vue from 'vue'; export const EventBus = new Vue();
Step 2: Create C1.vue file
<template> <div> <h1 style="color: #000">{{ data1 }}</h1> </div> </template> <script> import { EventBus } from "./EventBus.js"; export default { name: "C1", data() { return { data1: "C1 data", }; }, created() { EventBus.$on("listener-for-c1", (data) => { alert("C1 Event Listener Called!"); this.data1 = data; }); }, }; </script>
Step 3: Create C2.vue file
<template> <div> <h1>{{ data2 }}</h1> <button v-on:click="btnClicked">Click me to call c1 method</button> </div> </template> <script> import { EventBus } from "./EventBus.js"; export default { name: "C2", data() { return { data2: "C2 data", }; }, created() {}, methods: { btnClicked() { //We are calling here listener for C1 from C2 EventBus.$emit("listener-for-c1", this.data2); }, }, }; </script>
Bonus Point: To turn off the listener, use EventBus.$off() method of event bus. By the way, the listener is removed by the vue on the instance destruction but you can remove it using the $off method.